Suleman Ahmed - Blog Mastering JavaScript Arrays: A Comprehensive Guide
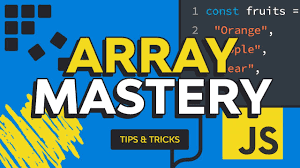
JavaScript arrays are the backbone of data manipulation and storage in web development. They provide a versatile and powerful way to work with collections of data, offering a myriad of methods and functionalities for efficient handling. In this article, we'll explore the fundamentals of JavaScript arrays, delve into their various features, and uncover best practices for mastering their usage.
Understanding JavaScript Arrays
At its core, an array in JavaScript is a special type of object used to store multiple values within a single variable. Arrays can contain elements of any data type, including numbers, strings, objects, functions, and even other arrays. They are defined using square brackets [ ]
and can be initialized empty or with initial values.
// Initializing an empty array
let myArray = [];
// Initializing an array with values
let fruits = ['apple', 'banana', 'orange'];
Accessing Array Elements
Array elements can be accessed using their index, starting from 0 for the first element. JavaScript also supports negative indexing, where -1
refers to the last element, -2
refers to the second last, and so on.
let fruits = ['apple', 'banana', 'orange'];
console.log(fruits[0]); // Output: 'apple'
console.log(fruits[2]); // Output: 'orange'
console.log(fruits[-1]); // Output: 'orange'
Array Methods for Manipulation
JavaScript provides a rich set of built-in methods for manipulating arrays, including adding or removing elements, iterating over arrays, sorting, filtering, and much more. Here are some commonly used array methods:
push()
: Adds one or more elements to the end of an array.pop()
: Removes the last element from an array and returns it.shift()
: Removes the first element from an array and returns it.unshift()
: Adds one or more elements to the beginning of an array.splice()
: Adds or removes elements from an array at a specified index.slice()
: Returns a shallow copy of a portion of an array.forEach()
: Executes a provided function once for each array element.map()
: Creates a new array by applying a function to each element of an existing array.filter()
: Creates a new array with elements that pass a test.sort()
: Sorts the elements of an array in place.reverse()
: Reverses the order of the elements in an array.
let numbers = [1, 2, 3, 4, 5];
// Adding elements
numbers.push(6);
console.log(numbers); // Output: [1, 2, 3, 4, 5, 6]
// Removing elements
numbers.pop();
console.log(numbers); // Output: [1, 2, 3, 4, 5]
Iterating Over Arrays
Iterating over arrays is a common task in JavaScript, and there are several methods to accomplish this, such as for
loops, forEach()
, map()
, filter()
, and reduce()
. Each method offers different functionalities and use cases, allowing developers to choose the most suitable approach based on their requirements.
let numbers = [1, 2, 3, 4, 5];
// Using forEach()
numbers.forEach(function(number) {
console.log(number);
});
// Using map()
let squaredNumbers = numbers.map(function(number) {
return number * number;
});
console.log(squaredNumbers); // Output: [1, 4, 9, 16, 25]
Conclusion
JavaScript arrays are a fundamental aspect of web development, providing a versatile and efficient means of working with collections of data. By understanding their fundamentals, accessing array elements, leveraging array methods for manipulation, and iterating over arrays effectively, developers can unlock the full potential of JavaScript arrays in their projects.
Mastering JavaScript arrays is essential for any developer looking to build dynamic and interactive web applications. By incorporating these concepts and best practices into your development workflow, you can streamline data handling, enhance code readability, and create robust and efficient solutions for a wide range of use cases.
So, dive into the world of JavaScript arrays, experiment with their features, and elevate your web development skills to new heights!
This article provides a comprehensive overview of JavaScript arrays, covering their fundamentals, manipulation methods, iteration techniques, and best practices. Whether you're a beginner learning the ropes or an experienced developer seeking to enhance your skills, mastering JavaScript arrays is a crucial step towards becoming proficient in web development.